Today, we want to share our experience in developing admin panels (CMS) using Filament, which is an incredibly powerful library of fullstack components based on the Laravel framework and Livewire technology.
We use Laravel as the primary framework for backend development in all our projects, and at some point, the question arose: which solution should we choose for admin panels? We decided on Filament.
We spent quite some time choosing between Filament and other admin panels. We'll try to explain why we believe Filament is perhaps the best choice you can make if your work involves writing CMS.
Why do you even need an admin panel?
We think it's well-known that no corporate website or online store can exist without a convenient content management tool. A powerful admin panel should provide tools that facilitate the creation, editing, and publishing of various types of content.
Filament is one of those tools that does not offer a ready-made solution. You have to create the necessary sections yourself, describe the fields and relationships for your entities.
Getting Started with Filament
Integrating Filament into your project is very simple. You just need to pull it in via composer and run the artisan command filament:install --panels, and then create an admin user with artisan make:filament-user.
Now, navigating to the /admin path, you will see an empty dashboard. This completes the minimal setup of Filament.
Sections (referred to as resources here), pages, themes, panels, widgets, and much more are also generated by the artisan make:filament-* commands, and then edited to meet the needs of the project.
Here are some cool features of Filament that definitely put it ahead of other admin panels.
No JS
You don't need to use JavaScript at all to construct pages. In Filament, all resource code is written in resource classes, in a declarative style in PHP, and the resource is conveniently divided into two parts, a form and a table, responsible for paginated display of all records and viewing/displaying individual records respectively. All necessary sorting and filtering are also defined in the resources, and this is done very simply.
In the code, it looks something like this:
public static function table(Table $table): Table
{
return $table
->columns([
Tables\Columns\TextColumn::make('name')
->searchable(isIndividual: true)
->sortable(),
Tables\Columns\TextColumn::make('email')
->label('Email address')
->searchable(isIndividual: true, isGlobal: false)
->sortable(),
Tables\Columns\TextColumn::make('country')
->getStateUsing(fn ($record): ?string => Country::find($record->addresses->first()?->country)?->name ?? null),
Tables\Columns\TextColumn::make('phone')
->searchable()
->sortable(),
])
->filters([
Tables\Filters\TrashedFilter::make(),
])
->actions([
Tables\Actions\EditAction::make(),
])
->groupedBulkActions([
Tables\Actions\DeleteBulkAction::make()
->action(function () {
Notification::make()
->title('Now, now, don\'t be cheeky, leave some records for others to play with!')
->warning()
->send();
}),
]);
}
And the result looks like this:
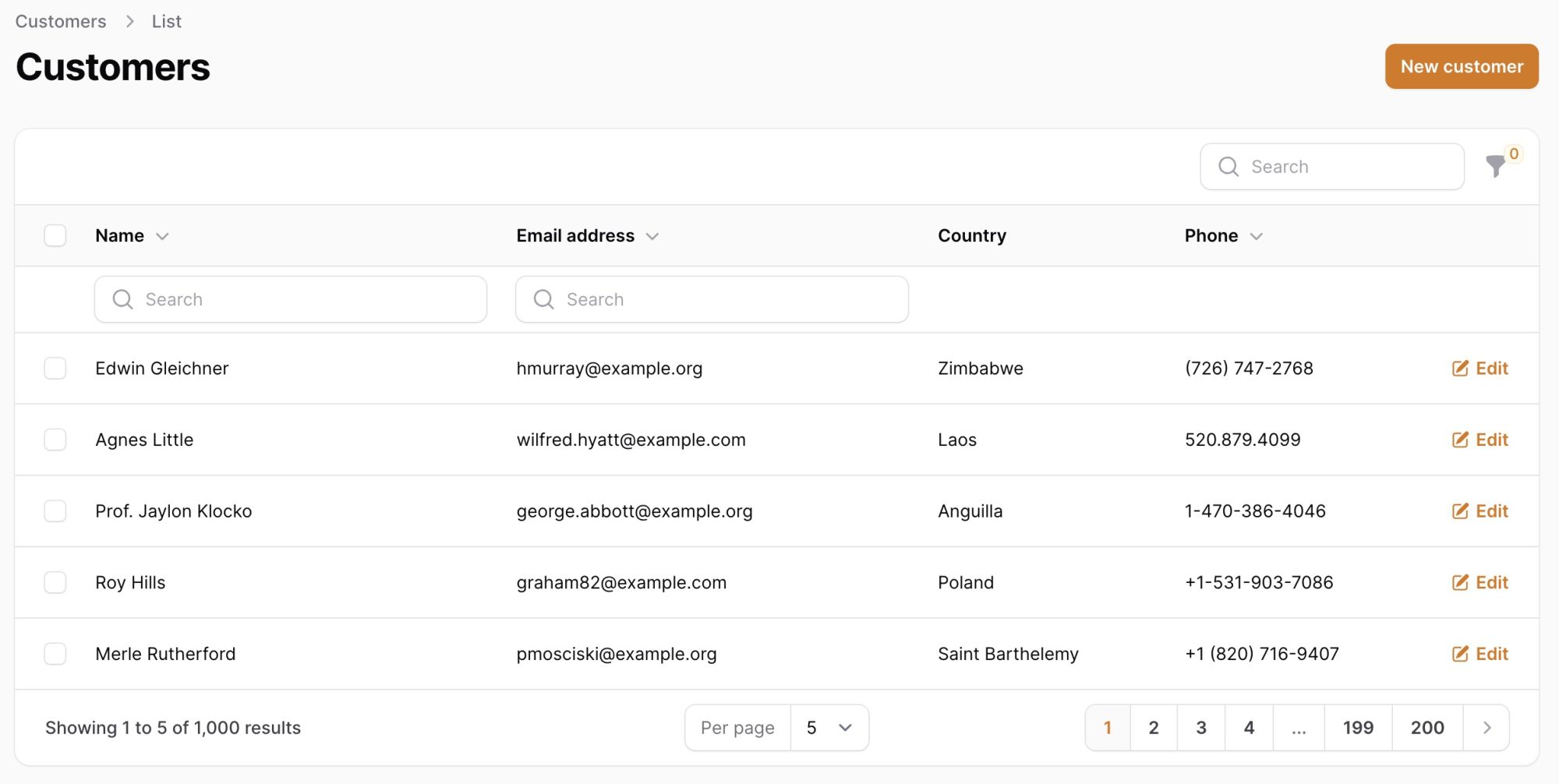
You can see that you can customize labels, make certain columns searchable, and also disable components, hide them, and perform other arbitrary manipulations based on some state. This makes the admin panel in Filament interactive as well.
If you feel that this is not enough, you can easily write custom JavaScript with any logic you need. The script can be either global (it will work on any page) or specific to a particular page.
Components
Filament is positioned as a set of beautiful and functional components. This allows you to significantly speed up the process of creating admin panels and focus on the application logic.
Forms with various field types and validation simplify the data entry process. Tables with sorting, filtering, and pagination capabilities are designed for convenient data display. Charts help visualize data, and control panels allow you to create informative dashboards.
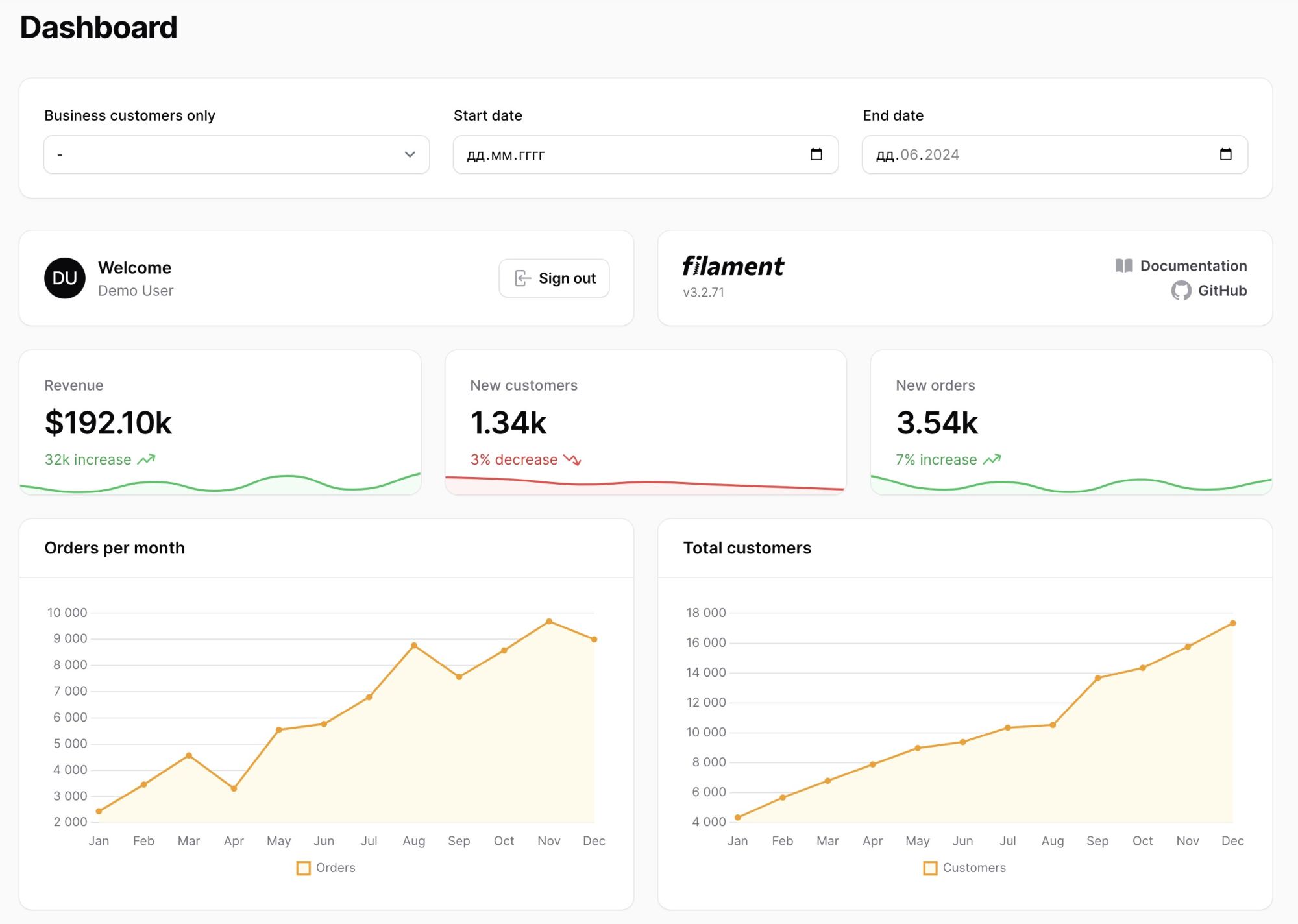
Access to Resources
A useful feature of Filament is its support for managing user access to various admin panel resources through Laravel policies. You simply describe the familiar policies for your models, and Filament resources automatically restrict access for users who do not meet the rules.
This allows you to flexibly configure user access rights.
Customization
Almost everything can be customized. From accent colors to page layouts using Livewire. You can cut out unnecessary functionality or, conversely, add missing features.
Scalability
Filament scales easily. You can write independent resources or entire admin panels within a single repository that are independent of each other. This means you don't have to worry about your code in one section breaking something in another.
Or you can maintain several versions of admin panels for users with different roles.
Filament also handles large amounts of data, complex forms, interactivity, and media management very easily. It's unlikely you'll encounter a situation where you can't do something in Filament, even if it's an undocumented feature.
Dependency Injection
In any method of any Filament component where a callback can be passed, you can modify the callback by declaring parameters that will be resolved by Filament based on the parameter name.
This way, you can pass $record into a callback, and this parameter will contain an instance of the model the form is working with. Thanks to this, you can, for example, check the values of the record fields.
Another option is the $get and $set parameters, which allow you to get or set the value of any field on the form at any time.
Active Developer Community
Filament is known for its large and active community that develops and supports it. This ensures the relevance of the library in the Laravel ecosystem and beyond.
Documentation and Learning Materials
Filament has quality documentation and numerous examples. Not everything is documented, but the community has released many articles covering various use cases. The documentation is easy to read and covers almost all aspects of working with the library.
To be honest, this wasn’t always the case, and it’s only from the third version of the library that the Filament maintainers have really focused on the documentation.
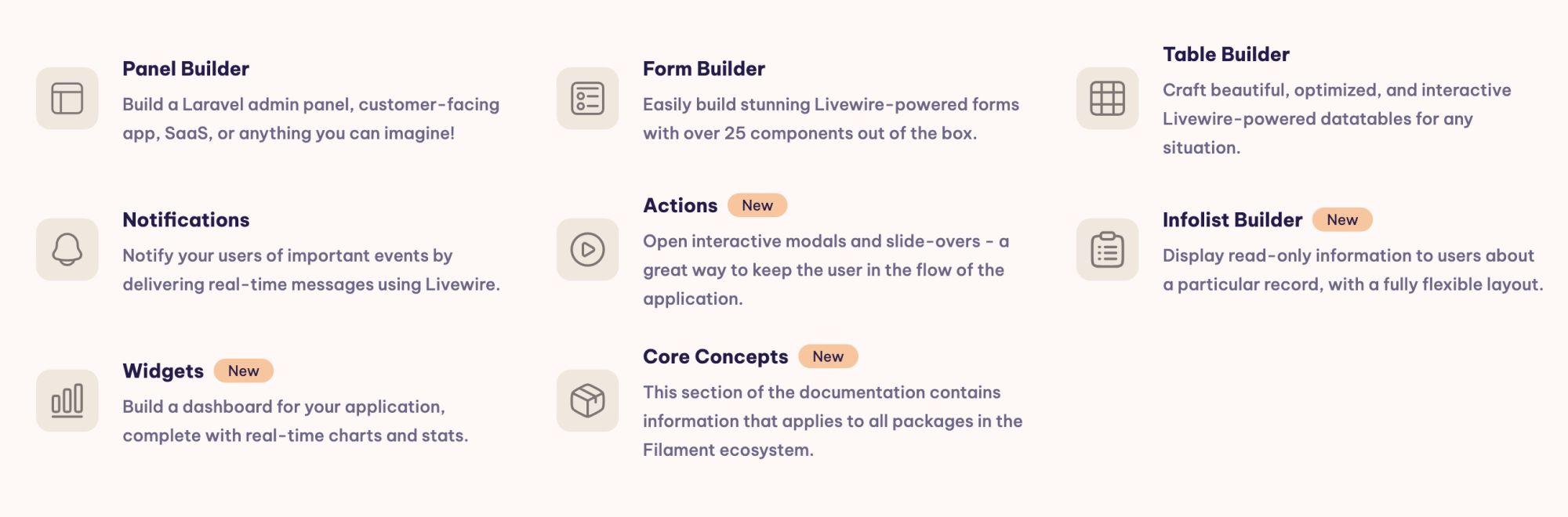
Conclusion
If you haven’t yet decided what to use for building admin panels for your projects or if you already have ongoing projects, we highly recommend trying Filament.